Final Project Ideas
Bereket Abraham
Andrew Ferg
Ryan Soussan
Lauren Berdick
Part I
Final Project Ideas
1. Smart food bin:
Problem: forgetting / not knowing how much food is left in your fridge
Possible solution: special bins for certain common foods that get used a lot. Use a (pressure?) sensor to detect how much is left. A computer will then send you alerts if you are running low. Or you can look up recipes based on what you have left.
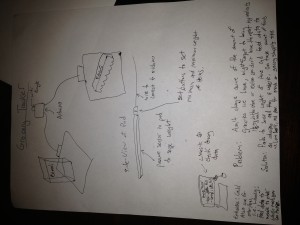
2. Automatic bartender:
Problem: the amount of liquid poured in each cup can vary a lot. We desire a system to pour standard, repeatable amounts into each cup.
Possible solution: A small reservoir tank and an arduino controlling a valve. Similar to a water cooler.
Possible solution: Same as above but now the cups travel on a convenient conveyer belt.
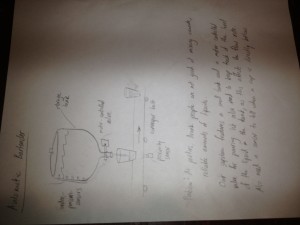
3. E-composer:
Problem: Writing music down on a score is annoying / difficult.
Possible Solution: create a computer program where you play an instrument and the computer transcribes the music.
4. E-composer II:
Problem: We want something to transcribe a music file, and break it into individual instrument parts.
Possible Solution: Some computer programs take in a music file and convert it into a score with notes. We just need to separate the individual instruments.
5. Smart light switch:
Problem: Hard to remember to turn off the light and leaving them on wastes electricity. It’s also annoying to turn off the lights from bed.
Possible Solution: Programmable light switch.
Possible solution: monitor a person’s light switch and then use machine learning to look for patterns. Similar to an implementation used for eco-friendly thermostats.
6. Indoor Directions:
Problem: It’s hard to make good directions for buildings and wall maps are annoying to use.
Possible Solution: Have a central screen where you input your destination. The lights in the floor will guide you to your destination. Your movements are tracked with (pressure/vibration?) sensors. Or just light up the entire path.
7. Shower Notes:
Problem: Can’t take notes during a shower.
Possible Solution: Create a waterproof iPad case. Create a way to use the touchscreen across the plastic casing. Indirect contact.
8. Networked Alarm Clock:
Problem: Several time keeping devices, including your online calendar, alarm clock, and cell phone.
Possible Solution: Program that interfaces mostly with google calendar. You can sleep until a set time or until your next class. Sets alarms on the clock and text/calls you on your phone.
9. Printed To Do List:
Problem: There are many good productivity tools online. How do they convert to the real world?
Possible Solution: Create printed post-it notes for each task or set of tasks.
10. Laundry folder
Problem: folding laundry is a pain.
Possible Solution: robot.
11. Breadboard Simulation / Avatar
Problem: Making circuits is hard.
Possible Solution: When using a breadboard, your parts are simulated on a computer. Computer can tell you stats on every wire/part.
12. Better oscilloscope
Problem: hard to use. Needs better interface.
13. Traveling Salesman
Problem: When you have a lot of destinations/errands, it is hard to plan the optimal route / order of events.
Possible Solution: event planner that takes into account opening/closing times, map directions, relative proximity, etc.
14. Digitizing notes
Problem: Lots of old notebooks are thrown away to save space.
Possible Solution: save your notes with some kind of automatic scanner. Enable searching.
15. Save energy
Problem: Several types of exercise can also produce energy.
Possible Solution: Attach a generator to a exercise bike, treadmill,
16. Dry shoes / socks
Problem: Canvas shoes and loafers get wet very easily. Uncomfortable.
Possible Solution: chemical treatment.
Possible Solution: Some kind of quick drying system.
17. Automatic tailor
Problem: Hard to find right size for online clothes shopping.
Possible Solution: special shirt / pants that finds your size.
18. Outlet finder
Problem: Hard to find a plug.
Possible Solution: detect radiation from AC current.
19. Bathroom finder.
Problem: Can never find the bathroom when you need it.
Possible Solution: App looks up the building plans and finds the nearest bathroom.
Possible Solution: tag bathroom with homing signal.
20. Lost Keys
Problem: Always losing your keys and other small objects.
Possible Solution: Close range tracker
21. Hologram
Problem: We don’t have holograms.
Possible Solution: use sound to set up standing waves. Trap gas in the nodes and shine a laser through it. Thus you get pixels.
22. Singing trainer
Problem: Hard to sing / embarrassed to practice in front of other people.
Possible Solution: program with feedback, scales, and other tools.
23. Music Updater
Problem: Hard to keep up to date with all of the musicians you like.
Possible Solution: Make an app that tracks whenever one of you artists releases a new song or album on Spotify or iTunes.
24. Outdoor refrigerator
Problem: During the winter, people heat their houses then cool them again to refrigerate food. Wastes energy.
Possible Solution: Pipe in outdoor air to cool the refrigerator. Somehow connect it to the outside world without cooling the rest of the house.
25. Robot Puppy
Problem: Usual robotic pets are stupid / unfeeling.
Possible Solution: Mod a robotic dog so that it recognizes faces and/or gestures.
26. Personalized ads
Problem: A lot of ads are not relevant.
Possible Solution: look at the clothes / other of a person to determine what types of ads to display.
27. Input automation
Problem: It’s annoying to do long sequences of clicks or enters on a computer, especially if you have to do it a lot of times.
Possible Solution: Have a program that monitors a sequences of clicks and turns them into an exe. Thus, you can simply a difficult or annoying interfaces into a series of chunks.
28. Commercial muter
Problem: TV commercials are annoying.
Possible Solution: Make a device/app that mutes ads and/or plays music. Maybe also include a short game during the commercial break.
29. Read while moving
Problem: It’s hard to read or watch movies while on the treadmill, bike, erg, etc.
Possible Solution: create a program that tracks your head and moves the display to match you.
Possible Solution: create a simple version of Google Glass to read books. Miniprojector / Mini screen?
30. Color Blind
Problem: People who are color blind might accidently mismatch clothes.
Possible Solution: Create an app that checks colors to make sure they are what you think they are. Or converts them into RGB numbers or sounds.
31. Artificial Synesthesia
Problem: It would be cool to experience colors / sounds in a new way.
Possible Solution: create a program that converts paintings into sounds or music into colors in an interesting way. Also leads to a new way to interface with music / painting.
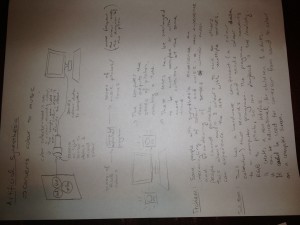
32. Regular laptop into touchscreen
Problem: Regular laptop is not a touchscreen.
Possible Solution: use an attachable sensor to detect either finger touches or a special pen on the screen, turning any normal screen into a touchscreen.
33. Auto Tie Tyer
Problem: When tying a tie, it’s hard to judge the proper length of the end ahead of time.
Possible Solution: program measures the tie and determines how much slack you should give to get the right length.
34. Noise cancelling
Problem: Sometimes you want to play an instrument late at night.
Possible Solution: Use the same tech as noise cancelling headphones. A device at the end of your instrument will exactly cancel out its noise. Only someone in its immediate vicinity can hear it.
35. LateX Math Handwriting Recognition
Problem: It’s annoying to use LaTeX – why can’t we just write math and digitize it? but computer-typeset math is very readable and modifiable. let’s build a system that lets you interact with a LaTeX document with just a hand-edited document.
36. Gesture control TV remote
Problem: this does not exist.
Solution: Wear gesture detecting device, i.e. a camera or kinect thing. Then hack a TV remote and use gestures to control a TV.
36. Gesture control bluetooth
Problem: It’s hard to easily move files.
Solution: Wear gesture detecting device, i.e. a camera or kinect thing. Then use gestures to move files between a computer and your wearable device (possibly with cloud storage).
36. Gesture control magnets
Problem: I do not have superpowers.
Solution: Wear gesture detecting device, i.e. a camera or flex sensors. Then use gestures to control electromagnets in your gloves.
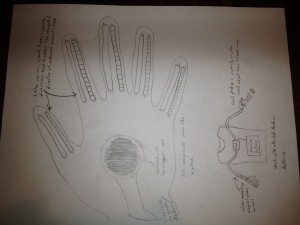
37. Surveillance Orb
Problem: It is difficult to monitor an entire room by looking at multiple camera feeds.
Solution: Stitch several cameras together and display them onto an orb (panoramic view). Then use control stick or gestures to move around in the room.
38. Surveillance Drone
Problem: Need to move to a different city, and need to apartment hunt.
Solution: Attach a camera to a robot (drone). Drive around the apartment.
39. Latex Checker
Problem: There’s no way to check the math in a latex document
Solution: Send the latex math to a math solver (like Mathematica) to make sure results are correct
40. Shortcut Recommender
Problem: Do lengthy or repetitive tasks inefficiently
Solution: Monitor computer use and automatically recommend shortcuts (keyboard, external buttons) for actions
41. Software/Hardware Recommender
Problem: We don’t know what efficient technology is available
Solution: Program to monitor computer use, recommend software and hardware devices that might help the user improve efficiency or generally improve their computing experience
42. Facial Hair Planner
Problem: Don’t know what facial hair would look awesome on face
Solution: Program to display different facial hair styles on image of user
43. Foot Pedal Interactor
Problem: We don’t use our feet when we use computers
Solution: Foot Pad/ Buttons to control actions on computer and games
44. Projectable Interactor
Problem: Some actions in software, games don’t map well to the keyboard
Solution: Have image projected on desktop, sense touches on the image and respond the the actions, change image/layout for each program
45. Guitar Ear Trainer
Problem: Hard to learn to play by ear, inefficient feedback or need a second person
Solution: Program to play notes on a guitar, listen to guitarist’s response and note mistakes and accuracy
46. Resistive Electronic Pen
Problem: Electronic pens don’t have any resistance / feedback.
Solution: Make a pen with a magnet in the tip. Underneath the surface will be an electromagnet. More strength = more attraction = more surface friction. Maybe different brushes are more resistive.
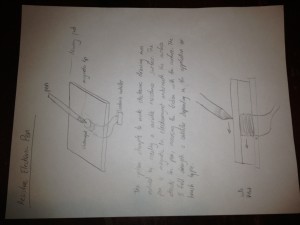
47. Repelling Electronic Pen
Problem: Electronic pens don’t have any resistance / feedback.
Solution: Same thing as above, but now the electromagnet is repelling. You would also have a way of forcing the electromagnet to be directly under the pen. Now, the pen will have a buffer directly over the surface that it cannot penetrate. But, you could push down with different strengths, which controls about an inch of z direction. Would make for a really interesting click or button.
48. Rotation Cubes
Problem: Its hard to rotate in 3D modeling or AutoCAD software.
Solution: A series of networked cubes. Each cube rotates and translates selected objects. A special cube can be assigned to rotate globally.
49. Thermal Beanie/other piece of clothing
Problem: Clothes don’t always keep you at the temperature you want
Solution: Have heat pads in clothing item that adjusts heat to keep you at desired temperature.
50. Magnetic Adjuster knob
Problem: Traditional slider knobs are boring / uninteresting.
Solution: Create a floating magnet suspended between two electromagnets. You can move the magnet up and down within the field, and the electromagnets will detect this and adjust accordingly.
51. Child Tracker
Problem: Nerve racking leaving a child alone in a room
Solution: Have the child wear a specific color and track them with cameras. Make sure they don’t go to dangerous areas.
Part II
Winner: Smart Food Bins
Why This Project: Out of our 51 ideas, this one had the most direct plan to carry it out, a very obvious utility, and easily fits within the budget. We liked the magnetic gloves and synesthesia idea as well, however, they gave difficult mechanical and creative concerns, respectively. The food pads had a clear cut implementation and utility that we felt we could create during the semester.
Part III
Smart Food Bins
Target user group: Very busy adults who have to cook for a household. This would most likely benefit families, where the parents often cook for three or more people, or roommates who cook for themselves, or co-ops who cook for a group. Their hectic lives mean that keeping track of food amounts is a very burdensome chore, and the fact that there are multiple people in the household means that it’s harder to keep track of what’s available since everyone is constantly eating and changing the amounts left. Their needs mainly center around adequately feeding their families. Their wants include saving time by reducing the number of trips to the grocery store and saving money by reducing waste.
The closest group we could find to model our target group is college students eating independently in large groups. This would include members of food cooperatives and residents of Spellman that cook together.
Problem Description: People are not always aware of the amount of groceries they have in their refrigerator or food pantry, especially when they are away from home. They might forget to buy certain items at the store and not be able to make certain recipes. Also, refrigerators and pantries can get very cluttered and disorganized. Thus, people may not be able to easily figure out they have left and what they are able to cook.
Possible Solution: We would make pads for food that would stream to a website the amount of each food that is available. We would make pads out of a durable, waterproof fabric with pressure sensors inside them to detect the food’s weight. The pads would communicate wirelessly over Bluetooth to an arduino, which would then stream data over wifi to a website. Users can login to the website to check the percent of each food that they still have. Users can label each pad on the website (i.e. eggs, milk, cereal) to indicate what it stores. The pads would have buttons to set (by measuring or by manual entry) their minimum and maximum weight, so that for example if a box of cereal is being used, the minimum weight would be the empty box, and the maximum weight would be the full box. The pads could come in different shapes and sizes.
An extension on the website could be a recipe checker, that allows the user to check what they still need in order to make a certain amount of servings for a dish. Additionally, the website could recommend dishes to make based on the ingredients that the user has.
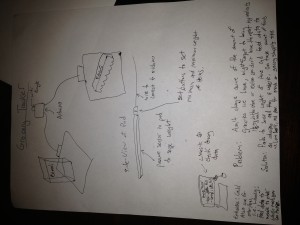
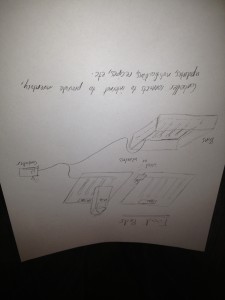